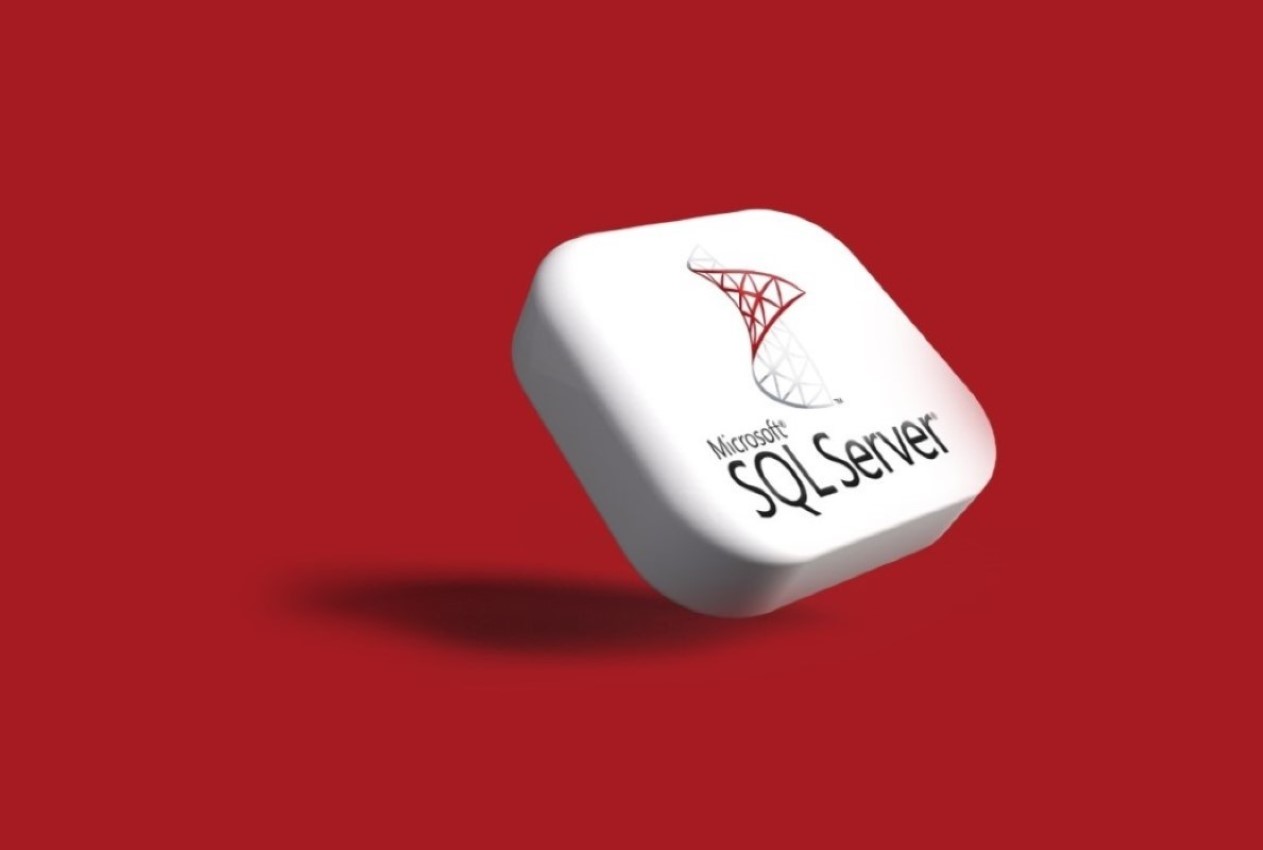
SQL is a powerful tool for managing and manipulating data, but it’s important to ensure that your SQL queries are optimized for performance. In this article, we will explore some tips and tricks for optimizing SQL queries and improving the performance of SQL statements.
One of the most important things to consider when optimizing SQL queries is the use of indexes. Indexes are a way to improve the performance of SQL queries by providing a faster way to locate and retrieve data. When creating an index, it’s important to choose the right columns to index and to use the appropriate index type. You can use the command “EXPLAIN” to understand how the database engine is executing the query and check if the indexes are being used or not.
EXPLAIN SELECT * FROM orders WHERE customer_id = 1;
Another important aspect of SQL performance tuning is the order of joins. When a query includes multiple tables, the order of joins can significantly impact performance. In general, it’s best to start with the smallest table and then join to the next largest table. This approach is called the “nested loop join” and it will help to minimize the number of rows that need to be scanned.
SELECT * FROM orders
JOIN customers ON orders.customer_id = customers.customer_id
JOIN products ON orders.product_id = products.product_id;
Limiting the number of rows returned by a query can also improve performance. In SQL, you can use the “LIMIT” clause to limit the number of rows returned by a query. This can be especially useful when working with large tables or when you only need a small subset of the data. For example, if you only need to retrieve the most recent 100 rows from a table, you can use the following query:
SELECT * FROM orders ORDER BY order_date DESC LIMIT 100;
Another important aspect of SQL performance tuning is the use of subqueries. Subqueries are a way to retrieve data from one table based on the values in another table. They can be useful for reducing the number of joins required in a query, but they can also be a performance bottleneck if not used carefully. It’s important to use subqueries only when necessary and to limit the number of rows returned by the subquery.
An important aspect of SQL performance tuning is the use of temporary tables. Temporary tables are a way to store the results of a query and use them in other queries. They can be useful for reducing the number of joins required in a query, but they can also be a performance bottleneck if not used carefully. It’s important to use temporary tables only when necessary and to limit the number of rows returned by the temporary table.
CREATE TEMPORARY TABLE temp_table AS (SELECT * FROM orders WHERE order_date >= '2022-01-01');
Another powerful feature of SQL is the ability to use Stored Procedures. Stored procedures are precompiled SQL statements that are stored in the database and can be called by the application. They can improve the performance of a query by reducing the amount of SQL code that needs to be sent to the database. When using stored procedures, it’s important to ensure that they are properly optimized and that the correct indexes are used.
CREATE PROCEDURE getOrdersByCustomer(IN customer_id INT)
BEGIN
SELECT * FROM orders WHERE customer_id = customer_id;
END;
Finally, it’s also important to keep in mind that SQL performance tuning is an ongoing process. As your data and usage patterns change, it’s important to continuously monitor and optimize your SQL queries.
SQL performance tuning is an important aspect of managing and manipulating data. By understanding the importance of indexes, join order, limiting rows, and the use of subqueries, you can ensure that your SQL queries are optimized for performance. Additionally, by using the command “EXPLAIN” you can understand how the database engine is executing the query and identify performance bottlenecks. With these tips and tricks, you can improve the performance of your SQL statements and extract insights from your data more efficiently.