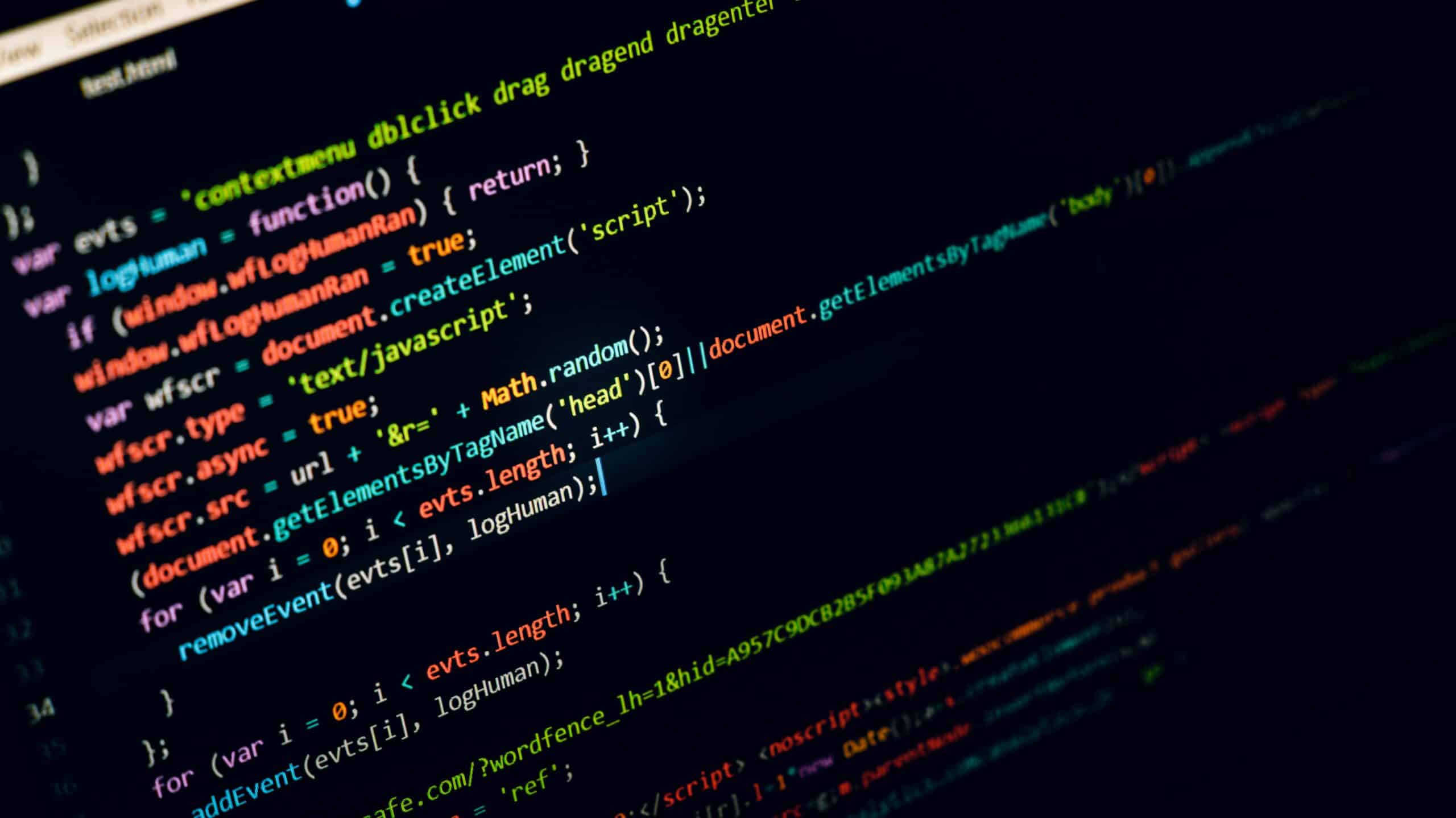
As a C# developer, there are certain functions that I find myself using on a regular basis. These functions can make my code more efficient, and more readable, or simply save me time when working on a project. In this blog post, I’d like to share with you my top ten most frequently used C# functions, along with some examples of how they can be used in a program.
1. string.Format()
– This function is useful for creating a formatted string using a specified format and a set of arguments. For example, consider the following code:
string name = "John";
int age = 30;
string output = string.Format("{0} is {1} years old.", name, age);
The output
the variable will contain the string “John is 30 years old.” The placeholders {0}
and {1}
in the format string are replaced with the corresponding arguments name
and age
.
2. int.TryParse()
– This function can be used to parse a string representation of a number to an integer and returns a boolean indicating whether the parse was successful. For example:
string input = "42";
int result;
if (int.TryParse(input, out result))
{
// Parsing was successful
}
else
{
// Parsing failed
}
3. Enum.TryParse()
– Similar to int.TryParse()
, this function can be used to parse a string representation of an enumeration value to an enumeration value. Here’s an example:
enum DaysOfTheWeek
{
Monday,
Tuesday,
Wednesday,
Thursday,
Friday,
Saturday,
Sunday
}
string input = "Friday";
DaysOfTheWeek day;
if (Enum.TryParse(input, out day))
{
// Parsing was successful
}
else
{
// Parsing failed
}
4. string.IsNullOrEmpty()
– This function is handy for checking whether a string is null
or an empty string. It returns a boolean value, which can be useful in an if statement or a ternary operator. For example:
string input = "";
if (string.IsNullOrEmpty(input))
{
// The string is either null or empty
}
else
{
// The string is not null or empty
}
6. string.Join()
– This function can be used to concatenate the elements of an array using a specified separator. For example:
string[] names = { "John", "Paul", "George", "Ringo" };
string output = string.Join(", ", names);
// The output string will be "John, Paul, George, Ringo"
6. Array.IndexOf()
– This function searches for a specified element in an array and returns the index of its first occurrence. If the element is not found, it returns -1. Here’s an example:
int[] numbers = { 1, 2, 3, 4, 5 };
int index = Array.IndexOf(numbers, 3);
// The index variable will be 2
7. List.Contains()
– This function is useful for determining whether a list contains a specific element. It returns a boolean value, which can be useful in an if statement or a ternary operator. For example:
List<string> names = new List<string> { "John", "Paul", "George", "Ringo" };
if (names.Contains("John"))
{
// The list contains the element "John"
}
else
{
// The list does not contain the element "John"
}
8. Math.Max()
– This function returns the larger of two values. It can be used with any data type that implements the IComparable
interface, including integers, doubles, and dates. For example:
int a = 10;
int b = 20;
int max = Math.Max(a, b);
// The max variable will be 20
9. Math.Min()
– This function returns the smaller of two values, and works in a similar way to Math.Max()
. For example:
double a = 3.14;
double b = 2.718;
double min = Math.Min(a, b);
// The min variable will be 2.718
10. string.Compare()
– This function compares two strings and returns an integer that indicates their relative position in the sort order. A value of 0 indicates that the strings are equal, a value less than 0 indicates that the first string comes before the second in the sort order, and a value greater than 0 indicates that the first string comes after the second in the sort order. For example:
string a = "apple";
string b = "banana";
int comparison = string.Compare(a, b);
// The comparison variable will be less than 0
I hope these examples have given you an idea of how these functions can be used in C# programs. Whether you’re a beginner or an experienced developer, I’m sure you’ll find these functions to be useful in your projects.